March 2022 Release (version 1.7.3) - Widgets! Built-in Terminal, Menu, and Built-in Editor Types
March 2022 Release (version 1.7.3)
Script Kit should auto-update or you can grab the downloads here: https://www.scriptkit.com/
Widgets - await widget()
A widget is a detached UI window that can control and listen to a script.
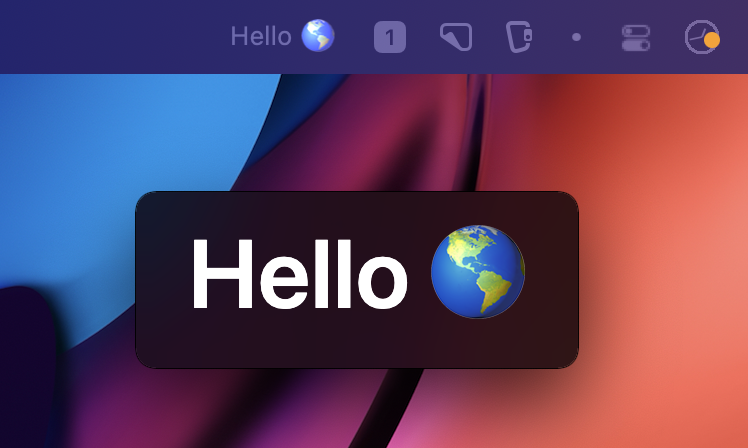
Open widget-hello-world in Script Kit
Widget Events
Open widget-events in Script Kit
Closing a Widget
There are 3 ways to close a widget:
- Hit "escape" with the widget focused
- End the process of the widget. Hit cmd+p with the main menu focused to see the running processes or
exit()
anywhere in the script. - Use a
ttl
(time to live) in the options when creating a widget
"Always on Top" and Locking the Widget
Open widget-always-on-top in Script Kit
With a widget focused, press cmd+l to "Lock" the widget. This will disable any possible mouse interactions (including moving, resizing, etc) and allow you to click through the widget to any windows underneath.
To "Unlock":
- three-fingered swipe up on OSX
- focus the widget
- hit cmd+l
You can now hit move, escape, etc the widget.
Built-in Terminal - await term()
term
is Script Kit's built-in terminal.
From the Main Menu
Type > into the main menu to open term
From a Script
Use the await term()
API to switch to the terminal.
Open term-hello-world in Script Kit
Note: If you want spawn a new Mac terminal, use
await terminal()
Pass Terminal Output to Script
If you end the terminal with cmd+enter, the script will continue and you can grab the latest text output from the terminal.
π: ctrl+any key will also end the terminal. This is a bug (it was only meant to be ctrl+c) which I'll fix soon. I'm also open to ideas for other shortcuts to "end" a terminal that aren't taken by vim/emacs/etc, because I know I'll be missing some. π:
term
doesn't grab keyboard focus when opening. I'll get that fixed ASAP!
Open term-returns in Script Kit
Menubar - menu()
menu
allows you to customize the menubar for Script Kit.
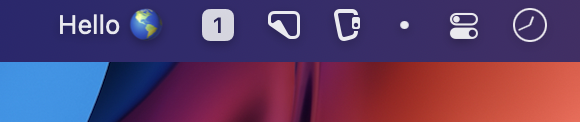
Open menu-hello-world in Script Kit
Menu with Scripts
The second arg of menu can be an array of scripts you wish to present in a drop-down menu. This way, on left-click, you'll get a list of scripts to pick from from the menubar rather than opening the main Script Kit UI.
Open menu-with-scripts in Script Kit
Built-in Editor Types
Script Kit's built-in editor now loads all of Script Kit's types! This was a huge undertaking that everyone just expects to work. You all know how that feels π
π: Please let me know if you see any missing. I noticed that I missed the types for
terminal
anditerm
when putting this post together π€¦ββοΈ.
March Plans
I'm dedicating March to DOCUMENTATION!!! (and bug-fixes)... I have a lot of script requests to follow-up on and work around the newsletter and other non-app stuff. I'm also moving this month, so y'all know how stressful that can be. So expect the April build to be extremely light feature-wise, but I will be set up in the new house ready to much more live-streaming and communication. Can't wait to share more! π
Questions?
I'm happy to help with any questions you may have!